To download an attachment, you must first find the download link and then redirect to another page.
The ending of the response from current page does not affect the functionalities on the second one.
using (SPSite site = new SPSite("http://SiteCollectionURL"))
{
using (SPWeb web = site.OpenWeb())
{
string file = string.Empty;
SPList list = web.Lists["MyList"];
SPListItem currentItem = myList.GetItemById(id);
if (currentItem["AttachmentName"] != null)
{
file = "/Lists/ MyList/Attachments/" +
id.ToString() + "/" +
currentItem["AttachmentName"].ToString();
System.Web.HttpContext.Current.Session["FileName"] =
currentItem["AttachmentName"].ToString();
System.Web.HttpContext.Current.Session["Attachment"] =
file.Trim();
}
else
{
lblReport.Text = "No File name found";
}
if (file != string.Empty)
{
Reponse.Redirect("download.aspx");
}
}
}
On the download.aspx page, you need to the code shown below to download the file.
if (System.Web.HttpContext.Current.Session["Attachment"] != null)
{
string strName = System.Web.HttpContext.Current.Session["FileName"].ToString();
string sbURL = System.Web.HttpContext.Current.Session["Attachment"].ToString();
System.Web.HttpResponse response;
response = System.Web.HttpContext.Current.Response;
System.Web.HttpContext.Current.Response.ContentEncoding =
System.Text.Encoding.Default;
response.AppendHeader("Content-disposition", "attachment; filename=" + strName);
response.AppendHeader("Pragma", "cache");
response.AppendHeader("Cache-control", "private");
response.Redirect(sbURL);
response.End();
}
Sunday 19 June 2011
Deleting an Attachment from SPList
Use below method to delete attachment of specific list item.
public void DeleteAttachment(int id,string fileName)
{
using (SPSite site = new SPSite("http://SiteCollectionURL"))
{
using (SPWeb web = site.OpenWeb())
{
SPList list = web.Lists["MyListName"];
SPListItem delItem = list.GetItemById(id);
SPAttachmentCollection files = delItem.Attachments;
files.Delete(fileName);
delItem.Update();
}
}
}
public void DeleteAttachment(int id,string fileName)
{
using (SPSite site = new SPSite("http://SiteCollectionURL"))
{
using (SPWeb web = site.OpenWeb())
{
SPList list = web.Lists["MyListName"];
SPListItem delItem = list.GetItemById(id);
SPAttachmentCollection files = delItem.Attachments;
files.Delete(fileName);
delItem.Update();
}
}
}
Adding an Attachment to an Item
Below code can be used to add attachment to list item.
using (SPSite site = new SPSite("http://SiteCollectionURL"))
{
using (SPWeb web = site.OpenWeb())
{
SPList list = web.Lists["MyListName"];
SPListItem newItem = list.GetItemById(id);
byte[] contents = null;
if (fileUpload.PostedFile != null && fileUpload.HasFile)
{
using (Stream fileStream = fileUpload.PostedFile.InputStream)
{
contents = new byte[fileStream.Length];
fileStream.Read(contents, 0, (int) fileStream.Length);
fileStream.Close();
}
SPAttachmentCollection attachments = newItem.Attachments;
string fileName = Path.GetFileName(fileUpload.PostedFile.FileName);
attachments.Add(fileName, contents);
newItem.Update();
}
}
}
using (SPSite site = new SPSite("http://SiteCollectionURL"))
{
using (SPWeb web = site.OpenWeb())
{
SPList list = web.Lists["MyListName"];
SPListItem newItem = list.GetItemById(id);
byte[] contents = null;
if (fileUpload.PostedFile != null && fileUpload.HasFile)
{
using (Stream fileStream = fileUpload.PostedFile.InputStream)
{
contents = new byte[fileStream.Length];
fileStream.Read(contents, 0, (int) fileStream.Length);
fileStream.Close();
}
SPAttachmentCollection attachments = newItem.Attachments;
string fileName = Path.GetFileName(fileUpload.PostedFile.FileName);
attachments.Add(fileName, contents);
newItem.Update();
}
}
}
Saturday 18 June 2011
How to check if User is Present in a Group
You can add extension method to SPUser class to achieve this requirement.
Add the below namespace.
using System.Linq;
public static bool UserExist(this SPUser user, string groupName)
{
return user.Groups.Cast().Any(gn => gn.Name == groupName);
}
Add the below namespace.
using System.Linq;
public static bool UserExist(this SPUser user, string groupName)
{
return user.Groups.Cast
}
How to close the sharepoint modal dialog and refresh the parent page.
Recently, I stumbled upon a situation where I had Calendar list and I had provided custom save and cancel button.
In List setting I had kept "Launch forms in a dialog" option to "No" so that my NewForm/EditForm/DispForm can open on same page instead of a dialog window.
But in case of Calendar View Edit and Display form always opens in Dialog.So after updating the record we explicitly need to close the dialog and redirect to any view.
At server side I tried below code.
Context.Response.Write("<script type='text/javascript'>window.frameElement.commitPopup();</script>");
Context.Response.Flush();
Context.Response.End();
But It didn't worke!!!! So I tried below approach and it worked.
Since I had to close the window only user is editing form Calendar view.So wherever you open a dialog a querystring is passed with name "IsDlg" and value ="1". So If ""IsDlg" is present in the URL redirect the user to your custom page from server side, and use below javascript to close the dialog.
Server Side Code
if (Request.QueryString["IsDlg"] != null)
{
dlgID = Request.QueryString["IsDlg"].ToString();
}
if (!string.IsNullOrEmpty(dlgID))
{
Response.Redirect(SPContext.Current.Web.Url + "/Default.aspx?Close=1");
}
Write below code in Default.aspx or your custom defualt page.
var closeFlag =location.search.substring(1).split("Close=");
if(closeFlag !="")
{
var flag = closeFlag[1].split("&");
if(flag=="1")
{
Close();
}
}
function Close()
{
// Will close the window and update the view.
window.frameElement.commitPopup();
}
</script>
In List setting I had kept "Launch forms in a dialog" option to "No" so that my NewForm/EditForm/DispForm can open on same page instead of a dialog window.
But in case of Calendar View Edit and Display form always opens in Dialog.So after updating the record we explicitly need to close the dialog and redirect to any view.
At server side I tried below code.
Context.Response.Write("<script type='text/javascript'>window.frameElement.commitPopup();</script>");
Context.Response.Flush();
Context.Response.End();
But It didn't worke!!!! So I tried below approach and it worked.
Since I had to close the window only user is editing form Calendar view.So wherever you open a dialog a querystring is passed with name "IsDlg" and value ="1". So If ""IsDlg" is present in the URL redirect the user to your custom page from server side, and use below javascript to close the dialog.
Server Side Code
if (Request.QueryString["IsDlg"] != null)
{
dlgID = Request.QueryString["IsDlg"].ToString();
}
if (!string.IsNullOrEmpty(dlgID))
{
Response.Redirect(SPContext.Current.Web.Url + "/Default.aspx?Close=1");
}
Write below code in Default.aspx or your custom defualt page.
var closeFlag =location.search.substring(1).split("Close=");
if(closeFlag !="")
{
var flag = closeFlag[1].split("&");
if(flag=="1")
{
Close();
}
}
function Close()
{
// Will close the window and update the view.
window.frameElement.commitPopup();
}
</script>
Tuesday 7 June 2011
Error while deploying solution
Error:
The specified path, file name, or both are too long. The fully qualified file name must be less than 260 characters, and the directory name must be less than 248 characters.
Solution:
Recently I encountered above error while deploying the solution. This error can be observed due to below two reasons:
1. Your Solution name/Project Name is too long
2. Your VS Solution/Project is under a deep folder structure for e.g. "D:\Dev\Code\Phase1\Latest\..\..\ etc..."
So to resolve this issue you can reduce the folder hierarchy or shorten the project/solution name.
The specified path, file name, or both are too long. The fully qualified file name must be less than 260 characters, and the directory name must be less than 248 characters.
Solution:
Recently I encountered above error while deploying the solution. This error can be observed due to below two reasons:
1. Your Solution name/Project Name is too long
2. Your VS Solution/Project is under a deep folder structure for e.g. "D:\Dev\Code\Phase1\Latest\..\..\ etc..."
So to resolve this issue you can reduce the folder hierarchy or shorten the project/solution name.
Sunday 5 June 2011
Adding Custom Links in Central Admin 2010
To Create a custom link in Central Admin 2010 below steps can be followed
1. Open Visual Studio 2010 and create "Empty SharePoint Project".
2. Add EmptyElement
3. Paste below XML
<?xml version="1.0" encoding="utf-8" ?>
<Elements xmlns="http://schemas.microsoft.com/sharepoint/">
<CustomAction Id="EA36F5EB-311D-4ee0-973E-1C503933D740"
GroupId="SiteCollections"
Location="Microsoft.SharePoint.Administration.Applications"
Sequence="1500"
Title="My Title"
Description="My Description">
<UrlAction Url="My URL" />
</CustomAction>
</Elements>
4. Add this Element in Feature.
5. Deploy it.
Note:
Above Link will appear under Central Admin -->Application Management-->Site Collections
To display the link at any other place "GroupId" and "Location" properties can be changed.
Please refer below table to get the Location and GroupID
1. Open Visual Studio 2010 and create "Empty SharePoint Project".
2. Add EmptyElement
3. Paste below XML
<?xml version="1.0" encoding="utf-8" ?>
<Elements xmlns="http://schemas.microsoft.com/sharepoint/">
<CustomAction Id="EA36F5EB-311D-4ee0-973E-1C503933D740"
GroupId="SiteCollections"
Location="Microsoft.SharePoint.Administration.Applications"
Sequence="1500"
Title="My Title"
Description="My Description">
<UrlAction Url="My URL" />
</CustomAction>
</Elements>
4. Add this Element in Feature.
5. Deploy it.
Note:
Above Link will appear under Central Admin -->Application Management-->Site Collections
To display the link at any other place "GroupId" and "Location" properties can be changed.
Please refer below table to get the Location and GroupID
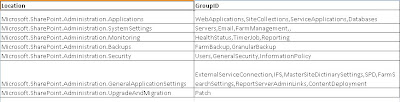
Subscribe to:
Posts (Atom)
Query List/Document Library in Specific Folder
To query SharePoint List or Document Library in specific Folder “ FolderServerRelativeUrl ” as part of the CAML Query Code Snippet ...
-
#Add a sharepoint group using power shell #Create a sharepoint custom permission using Power shell $web = get-SPWeb "http://sitecollect...
-
I am very sure that most of the Sharepoint developers must have used _spBodyOnLoadFunctionNames to inject javascript methods on the sharepo...
-
Recently I faced a javascript reference issue in my application. I had javascript file referenced in my master page. I had given relative pa...