Some time we have lots of heavy controls (like GridView,DataGrid) which emits lots of ViewState information on the page. This results to increase in Page size and apparently it would reduce the page performance.
So to reduce the page size ViewState can be stored on Server side which would reduce the page size.
We need to override SavePageStateToPersistenceMedium method to save the ViewState at server side and LoadPageStateFromPersistenceMedium method to retrieve the ViewState.
Note: These two methods needs to be overridden in code behind file of ASP.Net page.
Sample Code snippet.
protected override void SavePageStateToPersistenceMedium(object state)
{
try
{
string VSKey = "VIEWSTATE_" + base.Session.SessionID + "_" +
Request.RawUrl + "_" + DateTime.Now.Ticks.ToString();
Cache.Add(VSKey, state, null, DateTime.Now.AddMinutes(Session.Timeout),
Cache.NoSlidingExpiration, CacheItemPriority.Default, null);
ClientScript.RegisterHiddenField("__VIEWSTATE_KEY", VSKey);
}
catch
{
base.SavePageStateToPersistenceMedium(state);
}
}
protected override object LoadPageStateFromPersistenceMedium()
{
string VSKey = Request.Form["__VIEWSTATE_KEY"];
return Cache[VSKey];
}
Thursday 24 November 2011
Wednesday 3 August 2011
Admin SVC must be running in order to create deployment timer job
Issue : Recently, while using a PowerShell command (Update-SPSolution) I encountered below error.
Admin SVC must be running in order to create deployment timer job
Solution : You would encounter above error if “ SharePoint 2010 Administration” services is nor running. So to resolve above issue goto run -> type “services.msc” and start “SharePoint 2010 Administration” service.
Admin SVC must be running in order to create deployment timer job
Solution : You would encounter above error if “ SharePoint 2010 Administration” services is nor running. So to resolve above issue goto run -> type “services.msc” and start “SharePoint 2010 Administration” service.
Friday 1 July 2011
Multiple list instances of list definition
Problem:
Recently, I observed one weird thing in my SharePoint application.I was creating a list definition which had multiple views. I wanted to create one instance of the site definition but I could see multiple list instances of the same list in my site.
Reason:
I figured out the reason behind this issue.
In schema.xml under the views node I had multiple views definitions.
I had created one view and replicated the same for rest of the views.
<View DisplayName="By Customer Name" DefaultView="TRUE" BaseViewID="1" Type="HTML" MobileView="TRUE" MobileDefaultView="TRUE" ImageUrl="/_layouts/images/generic.png" XslLink="main.xsl" WebPartZoneID="Main" Url="By Customer Name.aspx" SetupPath="pages\viewpage.aspx">
<Toolbar Type="Standard" />
<XslLink>main.xsl</XslLink>
<Query>
</Query>
<ViewFields>
</ViewFields>
<RowLimit Paged="TRUE">100</RowLimit>
</View>
I was modifying the query and fieldrefs. Erroneously DefaultView="TRUE" attribute was present in all the views, which was resposible for creating multiple instances of the list and keeping that particular view as the default view.
Recently, I observed one weird thing in my SharePoint application.I was creating a list definition which had multiple views. I wanted to create one instance of the site definition but I could see multiple list instances of the same list in my site.
Reason:
I figured out the reason behind this issue.
In schema.xml under the views node I had multiple views definitions.
I had created one view and replicated the same for rest of the views.
<View DisplayName="By Customer Name" DefaultView="TRUE" BaseViewID="1" Type="HTML" MobileView="TRUE" MobileDefaultView="TRUE" ImageUrl="/_layouts/images/generic.png" XslLink="main.xsl" WebPartZoneID="Main" Url="By Customer Name.aspx" SetupPath="pages\viewpage.aspx">
<Toolbar Type="Standard" />
<XslLink>main.xsl</XslLink>
<Query>
</Query>
<ViewFields>
</ViewFields>
<RowLimit Paged="TRUE">100</RowLimit>
</View>
I was modifying the query and fieldrefs. Erroneously DefaultView="TRUE" attribute was present in all the views, which was resposible for creating multiple instances of the list and keeping that particular view as the default view.
Sunday 19 June 2011
How to programmatically download attachments from list items
To download an attachment, you must first find the download link and then redirect to another page.
The ending of the response from current page does not affect the functionalities on the second one.
using (SPSite site = new SPSite("http://SiteCollectionURL"))
{
using (SPWeb web = site.OpenWeb())
{
string file = string.Empty;
SPList list = web.Lists["MyList"];
SPListItem currentItem = myList.GetItemById(id);
if (currentItem["AttachmentName"] != null)
{
file = "/Lists/ MyList/Attachments/" +
id.ToString() + "/" +
currentItem["AttachmentName"].ToString();
System.Web.HttpContext.Current.Session["FileName"] =
currentItem["AttachmentName"].ToString();
System.Web.HttpContext.Current.Session["Attachment"] =
file.Trim();
}
else
{
lblReport.Text = "No File name found";
}
if (file != string.Empty)
{
Reponse.Redirect("download.aspx");
}
}
}
On the download.aspx page, you need to the code shown below to download the file.
if (System.Web.HttpContext.Current.Session["Attachment"] != null)
{
string strName = System.Web.HttpContext.Current.Session["FileName"].ToString();
string sbURL = System.Web.HttpContext.Current.Session["Attachment"].ToString();
System.Web.HttpResponse response;
response = System.Web.HttpContext.Current.Response;
System.Web.HttpContext.Current.Response.ContentEncoding =
System.Text.Encoding.Default;
response.AppendHeader("Content-disposition", "attachment; filename=" + strName);
response.AppendHeader("Pragma", "cache");
response.AppendHeader("Cache-control", "private");
response.Redirect(sbURL);
response.End();
}
The ending of the response from current page does not affect the functionalities on the second one.
using (SPSite site = new SPSite("http://SiteCollectionURL"))
{
using (SPWeb web = site.OpenWeb())
{
string file = string.Empty;
SPList list = web.Lists["MyList"];
SPListItem currentItem = myList.GetItemById(id);
if (currentItem["AttachmentName"] != null)
{
file = "/Lists/ MyList/Attachments/" +
id.ToString() + "/" +
currentItem["AttachmentName"].ToString();
System.Web.HttpContext.Current.Session["FileName"] =
currentItem["AttachmentName"].ToString();
System.Web.HttpContext.Current.Session["Attachment"] =
file.Trim();
}
else
{
lblReport.Text = "No File name found";
}
if (file != string.Empty)
{
Reponse.Redirect("download.aspx");
}
}
}
On the download.aspx page, you need to the code shown below to download the file.
if (System.Web.HttpContext.Current.Session["Attachment"] != null)
{
string strName = System.Web.HttpContext.Current.Session["FileName"].ToString();
string sbURL = System.Web.HttpContext.Current.Session["Attachment"].ToString();
System.Web.HttpResponse response;
response = System.Web.HttpContext.Current.Response;
System.Web.HttpContext.Current.Response.ContentEncoding =
System.Text.Encoding.Default;
response.AppendHeader("Content-disposition", "attachment; filename=" + strName);
response.AppendHeader("Pragma", "cache");
response.AppendHeader("Cache-control", "private");
response.Redirect(sbURL);
response.End();
}
Deleting an Attachment from SPList
Use below method to delete attachment of specific list item.
public void DeleteAttachment(int id,string fileName)
{
using (SPSite site = new SPSite("http://SiteCollectionURL"))
{
using (SPWeb web = site.OpenWeb())
{
SPList list = web.Lists["MyListName"];
SPListItem delItem = list.GetItemById(id);
SPAttachmentCollection files = delItem.Attachments;
files.Delete(fileName);
delItem.Update();
}
}
}
public void DeleteAttachment(int id,string fileName)
{
using (SPSite site = new SPSite("http://SiteCollectionURL"))
{
using (SPWeb web = site.OpenWeb())
{
SPList list = web.Lists["MyListName"];
SPListItem delItem = list.GetItemById(id);
SPAttachmentCollection files = delItem.Attachments;
files.Delete(fileName);
delItem.Update();
}
}
}
Adding an Attachment to an Item
Below code can be used to add attachment to list item.
using (SPSite site = new SPSite("http://SiteCollectionURL"))
{
using (SPWeb web = site.OpenWeb())
{
SPList list = web.Lists["MyListName"];
SPListItem newItem = list.GetItemById(id);
byte[] contents = null;
if (fileUpload.PostedFile != null && fileUpload.HasFile)
{
using (Stream fileStream = fileUpload.PostedFile.InputStream)
{
contents = new byte[fileStream.Length];
fileStream.Read(contents, 0, (int) fileStream.Length);
fileStream.Close();
}
SPAttachmentCollection attachments = newItem.Attachments;
string fileName = Path.GetFileName(fileUpload.PostedFile.FileName);
attachments.Add(fileName, contents);
newItem.Update();
}
}
}
using (SPSite site = new SPSite("http://SiteCollectionURL"))
{
using (SPWeb web = site.OpenWeb())
{
SPList list = web.Lists["MyListName"];
SPListItem newItem = list.GetItemById(id);
byte[] contents = null;
if (fileUpload.PostedFile != null && fileUpload.HasFile)
{
using (Stream fileStream = fileUpload.PostedFile.InputStream)
{
contents = new byte[fileStream.Length];
fileStream.Read(contents, 0, (int) fileStream.Length);
fileStream.Close();
}
SPAttachmentCollection attachments = newItem.Attachments;
string fileName = Path.GetFileName(fileUpload.PostedFile.FileName);
attachments.Add(fileName, contents);
newItem.Update();
}
}
}
Saturday 18 June 2011
How to check if User is Present in a Group
You can add extension method to SPUser class to achieve this requirement.
Add the below namespace.
using System.Linq;
public static bool UserExist(this SPUser user, string groupName)
{
return user.Groups.Cast().Any(gn => gn.Name == groupName);
}
Add the below namespace.
using System.Linq;
public static bool UserExist(this SPUser user, string groupName)
{
return user.Groups.Cast
}
How to close the sharepoint modal dialog and refresh the parent page.
Recently, I stumbled upon a situation where I had Calendar list and I had provided custom save and cancel button.
In List setting I had kept "Launch forms in a dialog" option to "No" so that my NewForm/EditForm/DispForm can open on same page instead of a dialog window.
But in case of Calendar View Edit and Display form always opens in Dialog.So after updating the record we explicitly need to close the dialog and redirect to any view.
At server side I tried below code.
Context.Response.Write("<script type='text/javascript'>window.frameElement.commitPopup();</script>");
Context.Response.Flush();
Context.Response.End();
But It didn't worke!!!! So I tried below approach and it worked.
Since I had to close the window only user is editing form Calendar view.So wherever you open a dialog a querystring is passed with name "IsDlg" and value ="1". So If ""IsDlg" is present in the URL redirect the user to your custom page from server side, and use below javascript to close the dialog.
Server Side Code
if (Request.QueryString["IsDlg"] != null)
{
dlgID = Request.QueryString["IsDlg"].ToString();
}
if (!string.IsNullOrEmpty(dlgID))
{
Response.Redirect(SPContext.Current.Web.Url + "/Default.aspx?Close=1");
}
Write below code in Default.aspx or your custom defualt page.
var closeFlag =location.search.substring(1).split("Close=");
if(closeFlag !="")
{
var flag = closeFlag[1].split("&");
if(flag=="1")
{
Close();
}
}
function Close()
{
// Will close the window and update the view.
window.frameElement.commitPopup();
}
</script>
In List setting I had kept "Launch forms in a dialog" option to "No" so that my NewForm/EditForm/DispForm can open on same page instead of a dialog window.
But in case of Calendar View Edit and Display form always opens in Dialog.So after updating the record we explicitly need to close the dialog and redirect to any view.
At server side I tried below code.
Context.Response.Write("<script type='text/javascript'>window.frameElement.commitPopup();</script>");
Context.Response.Flush();
Context.Response.End();
But It didn't worke!!!! So I tried below approach and it worked.
Since I had to close the window only user is editing form Calendar view.So wherever you open a dialog a querystring is passed with name "IsDlg" and value ="1". So If ""IsDlg" is present in the URL redirect the user to your custom page from server side, and use below javascript to close the dialog.
Server Side Code
if (Request.QueryString["IsDlg"] != null)
{
dlgID = Request.QueryString["IsDlg"].ToString();
}
if (!string.IsNullOrEmpty(dlgID))
{
Response.Redirect(SPContext.Current.Web.Url + "/Default.aspx?Close=1");
}
Write below code in Default.aspx or your custom defualt page.
var closeFlag =location.search.substring(1).split("Close=");
if(closeFlag !="")
{
var flag = closeFlag[1].split("&");
if(flag=="1")
{
Close();
}
}
function Close()
{
// Will close the window and update the view.
window.frameElement.commitPopup();
}
</script>
Tuesday 7 June 2011
Error while deploying solution
Error:
The specified path, file name, or both are too long. The fully qualified file name must be less than 260 characters, and the directory name must be less than 248 characters.
Solution:
Recently I encountered above error while deploying the solution. This error can be observed due to below two reasons:
1. Your Solution name/Project Name is too long
2. Your VS Solution/Project is under a deep folder structure for e.g. "D:\Dev\Code\Phase1\Latest\..\..\ etc..."
So to resolve this issue you can reduce the folder hierarchy or shorten the project/solution name.
The specified path, file name, or both are too long. The fully qualified file name must be less than 260 characters, and the directory name must be less than 248 characters.
Solution:
Recently I encountered above error while deploying the solution. This error can be observed due to below two reasons:
1. Your Solution name/Project Name is too long
2. Your VS Solution/Project is under a deep folder structure for e.g. "D:\Dev\Code\Phase1\Latest\..\..\ etc..."
So to resolve this issue you can reduce the folder hierarchy or shorten the project/solution name.
Sunday 5 June 2011
Adding Custom Links in Central Admin 2010
To Create a custom link in Central Admin 2010 below steps can be followed
1. Open Visual Studio 2010 and create "Empty SharePoint Project".
2. Add EmptyElement
3. Paste below XML
<?xml version="1.0" encoding="utf-8" ?>
<Elements xmlns="http://schemas.microsoft.com/sharepoint/">
<CustomAction Id="EA36F5EB-311D-4ee0-973E-1C503933D740"
GroupId="SiteCollections"
Location="Microsoft.SharePoint.Administration.Applications"
Sequence="1500"
Title="My Title"
Description="My Description">
<UrlAction Url="My URL" />
</CustomAction>
</Elements>
4. Add this Element in Feature.
5. Deploy it.
Note:
Above Link will appear under Central Admin -->Application Management-->Site Collections
To display the link at any other place "GroupId" and "Location" properties can be changed.
Please refer below table to get the Location and GroupID
1. Open Visual Studio 2010 and create "Empty SharePoint Project".
2. Add EmptyElement
3. Paste below XML
<?xml version="1.0" encoding="utf-8" ?>
<Elements xmlns="http://schemas.microsoft.com/sharepoint/">
<CustomAction Id="EA36F5EB-311D-4ee0-973E-1C503933D740"
GroupId="SiteCollections"
Location="Microsoft.SharePoint.Administration.Applications"
Sequence="1500"
Title="My Title"
Description="My Description">
<UrlAction Url="My URL" />
</CustomAction>
</Elements>
4. Add this Element in Feature.
5. Deploy it.
Note:
Above Link will appear under Central Admin -->Application Management-->Site Collections
To display the link at any other place "GroupId" and "Location" properties can be changed.
Please refer below table to get the Location and GroupID
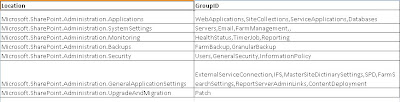
Wednesday 25 May 2011
How to check your code is running under sandbox Worker Process
You can use below code to check if your application is running withing sandbox worker process
if(System.AppDomain.CurrentDomain.FriendlyName.Contains(“Sandbox”))
{
// Your code is running in the sandbox.
}
if(System.AppDomain.CurrentDomain.FriendlyName.Contains(“Sandbox”))
{
// Your code is running in the sandbox.
}
Monday 21 February 2011
Using ~SiteCollection prefix for referring javascript files
Recently I faced a javascript reference issue in my application. I had javascript file referenced in my master page. I had given relative path to the JavaScript files.
<span style="font-weight:bold;"><script type="text/javascript" src= "../../Script%20Library/jquery-1.4.2.min.js"></script></span>
It was not working for all the pages across application due to different hierarchy.
So below dynamic reference style can be used to overcome this issue.
<script type="text/javascript" src= "<asp:Literal runat='server' Text='<%$SPUrl:~sitecollection/Script%20Library/jquery-1.4.2.min.js%>'/>"></script></span>
<span style="font-weight:bold;"><script type="text/javascript" src= "../../Script%20Library/jquery-1.4.2.min.js"></script></span>
It was not working for all the pages across application due to different hierarchy.
So below dynamic reference style can be used to overcome this issue.
<script type="text/javascript" src= "<asp:Literal runat='server' Text='<%$SPUrl:~sitecollection/Script%20Library/jquery-1.4.2.min.js%>'/>"></script></span>
Wednesday 26 January 2011
Add/remove sharepoint group using power shell
#Add a sharepoint group using power shell
#Create a sharepoint custom permission using Power shell
$web = get-SPWeb "http://sitecollectionName"
$member = $web.AllUsers["domain\username"]
$CustomPermission ="Permission Name"
$CustomRoleRoledef = New-Object "Microsoft.SharePoint.SPRoleDefinition"
$CustomRoleRoledef.Name = "$CustomPermission"
$CustomRoleRoledef.Description = "Custom permission level for Site Owners"
$CustomRoleRoledef.BasePermissions =
"CancelCheckout,AddListItems,EditListItems,DeleteListItems,ViewListItems,OpenItems,ViewVersions,DeleteVersions,CreateAlerts,ViewFormPages,ViewUsageData,ViewPages,BrowseUserInfo,ManageAlerts,UseRemoteAPIs,UseClientIntegration,Open"
$web.RoleDefinitions.Add($CustomRoleRoledef)
$web.Update()
# Create custom group
$CustomGroup ="My Custom Group"
$web.SiteGroups.Add("$CustomGroup", $member, $null,"Custom Group ")
#Assign Permission to the Group
$customPermission = $web.RoleDefinitions["$CustomRoleRoledef "]
# If you don't want to use custom permission, you can directly give existing permission name i.e "Full Control/Contributor/Design/Visitor"
#$existingPermission = $web.RoleDefinitions["Design"]
$customPermissionAssignment = New-Object "Microsoft.SharePoint.SPRoleAssignment" -ArgumentList $web.SiteGroups["$CustomGroup"]
$customPermissionAssignment.RoleDefinitionBindings.Add($customPermission)
$web.RoleAssignments.Add($techAdminRoleAssignment)
#Remove a sharepoint group using power shell
#Remove Groups if already exist
$GroupName ="Custom Group"
$objSiteGroup = $web.SiteGroups["$GroupName"]
if ($objSiteGroup)
{
$web.SiteGroups.Remove("$GroupName")
}
Note: you can save above script in .ps1 extension file and execute in power shell
#Create a sharepoint custom permission using Power shell
$web = get-SPWeb "http://sitecollectionName"
$member = $web.AllUsers["domain\username"]
$CustomPermission ="Permission Name"
$CustomRoleRoledef = New-Object "Microsoft.SharePoint.SPRoleDefinition"
$CustomRoleRoledef.Name = "$CustomPermission"
$CustomRoleRoledef.Description = "Custom permission level for Site Owners"
$CustomRoleRoledef.BasePermissions =
"CancelCheckout,AddListItems,EditListItems,DeleteListItems,ViewListItems,OpenItems,ViewVersions,DeleteVersions,CreateAlerts,ViewFormPages,ViewUsageData,ViewPages,BrowseUserInfo,ManageAlerts,UseRemoteAPIs,UseClientIntegration,Open"
$web.RoleDefinitions.Add($CustomRoleRoledef)
$web.Update()
# Create custom group
$CustomGroup ="My Custom Group"
$web.SiteGroups.Add("$CustomGroup", $member, $null,"Custom Group ")
#Assign Permission to the Group
$customPermission = $web.RoleDefinitions["$CustomRoleRoledef "]
# If you don't want to use custom permission, you can directly give existing permission name i.e "Full Control/Contributor/Design/Visitor"
#$existingPermission = $web.RoleDefinitions["Design"]
$customPermissionAssignment = New-Object "Microsoft.SharePoint.SPRoleAssignment" -ArgumentList $web.SiteGroups["$CustomGroup"]
$customPermissionAssignment.RoleDefinitionBindings.Add($customPermission)
$web.RoleAssignments.Add($techAdminRoleAssignment)
#Remove a sharepoint group using power shell
#Remove Groups if already exist
$GroupName ="Custom Group"
$objSiteGroup = $web.SiteGroups["$GroupName"]
if ($objSiteGroup)
{
$web.SiteGroups.Remove("$GroupName")
}
Note: you can save above script in .ps1 extension file and execute in power shell
Monday 24 January 2011
The Server Returned a non-specific error when trying to get data from the data source
This issue occurs when you do backup restore of the site/site collection. Due to this you can not see the page in design mode due to some reference changes and will not be able to add any new data sources on the page.
To resolve this issue follow below steps.
1. Go to Insert - New Item Form
2. Click on Custom List Form
3. Choose your custom list.
4. Choose form type (new/edit/display).
5. Select the "DataSources" section of the newly created dataformwebpart.
6. Now replace the existing "Datasources" section with selected one.
7. Remove the old DataFormweb part.
Just after saving the form mentioned error should go and you should be able to see all the controls with existing binding.
To resolve this issue follow below steps.
1. Go to Insert - New Item Form
2. Click on Custom List Form
3. Choose your custom list.
4. Choose form type (new/edit/display).
5. Select the "DataSources" section of the newly created dataformwebpart.
6. Now replace the existing "Datasources" section with selected one.
7. Remove the old DataFormweb part.
Just after saving the form mentioned error should go and you should be able to see all the controls with existing binding.
Subscribe to:
Posts (Atom)
Query List/Document Library in Specific Folder
To query SharePoint List or Document Library in specific Folder “ FolderServerRelativeUrl ” as part of the CAML Query Code Snippet ...
-
#Add a sharepoint group using power shell #Create a sharepoint custom permission using Power shell $web = get-SPWeb "http://sitecollect...
-
I am very sure that most of the Sharepoint developers must have used _spBodyOnLoadFunctionNames to inject javascript methods on the sharepo...
-
Recently I faced a javascript reference issue in my application. I had javascript file referenced in my master page. I had given relative pa...